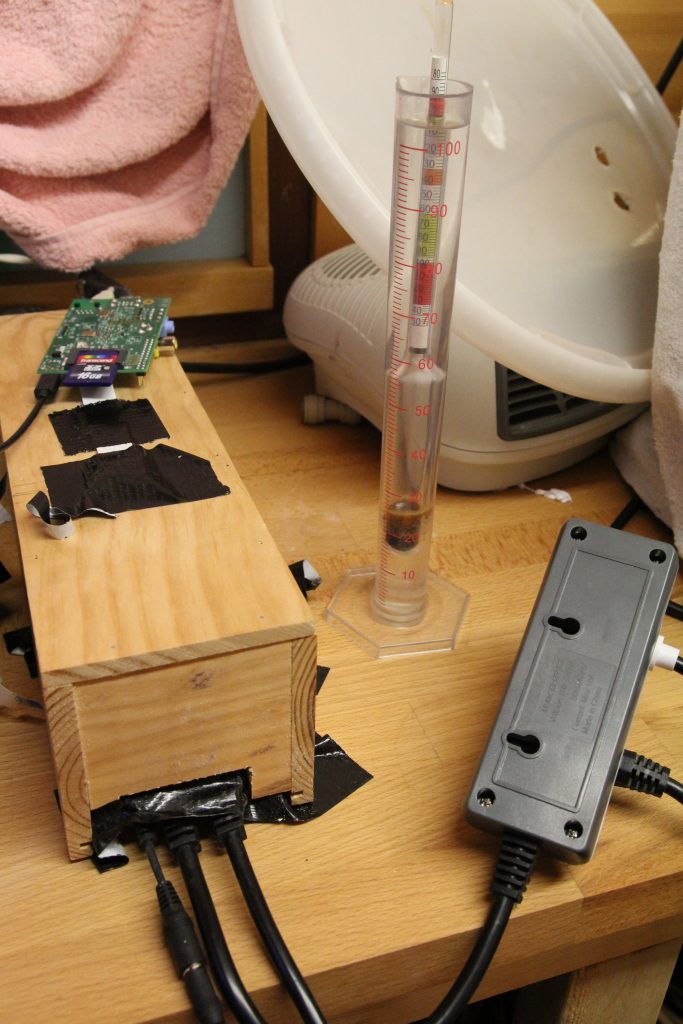
Beer chiller to cool the fermentation
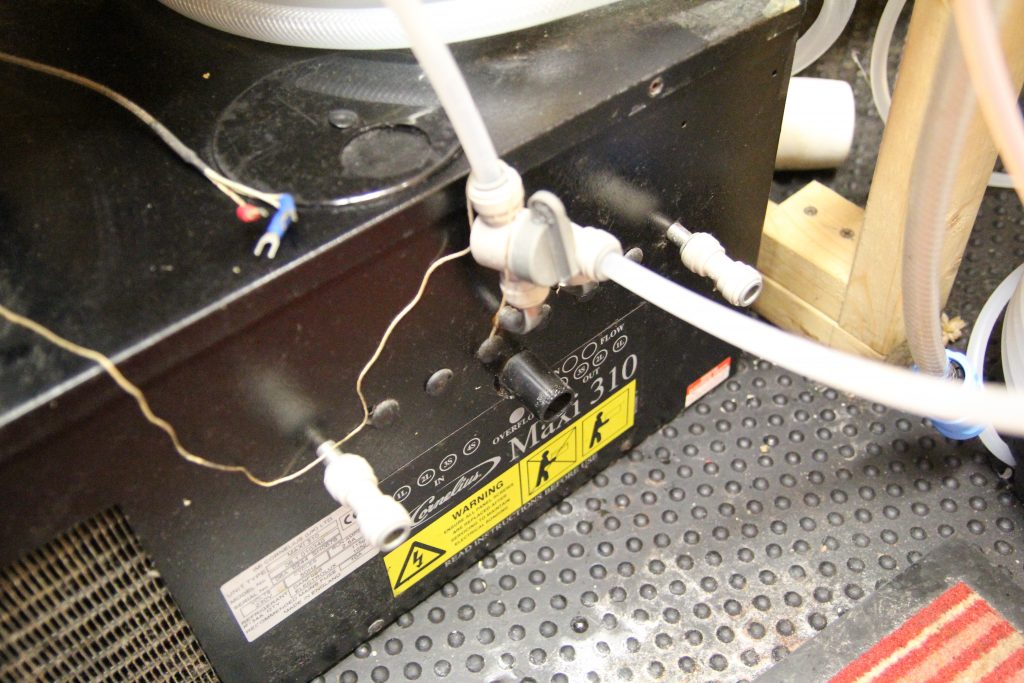
Parasene soil warming cable to warm the fermentation (75W) along with SS coil to cool it, attached to beer chiller
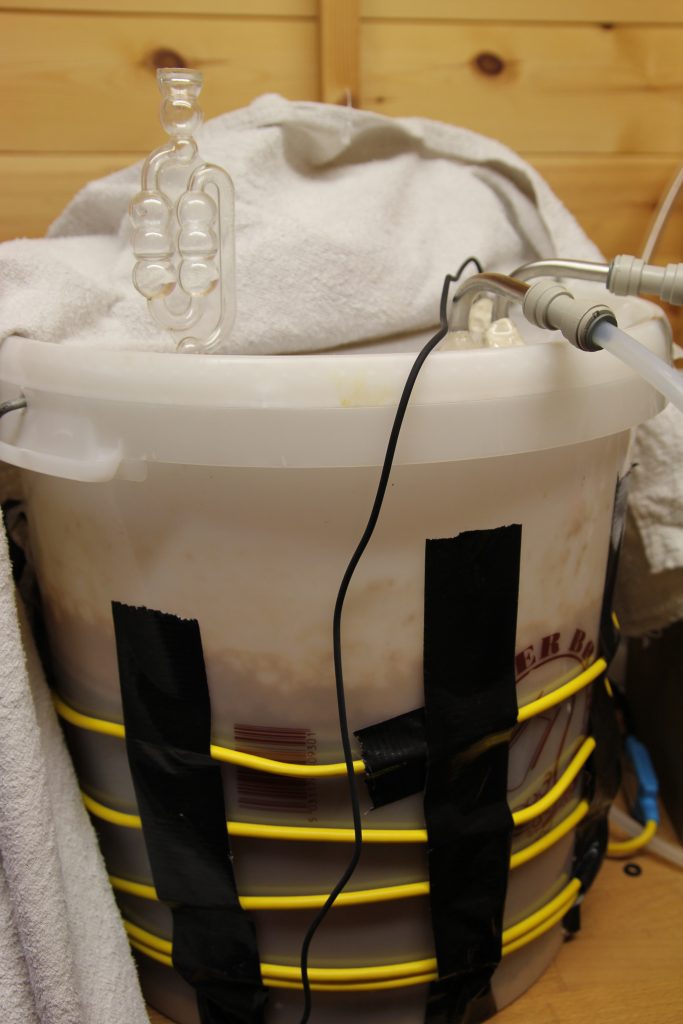
Sourcecode to generate a CSV file of temperatures
#!/bin/bash
while true; do
# Take photo using current date
DATE=$(date +"%Y-%m-%d-%H%M")
raspistill -vf -hf -o $DATE.jpg
ARRAY=()
X=0
# Try different thresholds
for I in 90 80 70 60 50 40 30 20 10; do
# Ensure there is at least PIX pixels
for PIX in 50 20 5; do
# Shear image by different amounts
for SHEAR in 65 10; do
Z=$(../../ssocr/ssocr -d3 -i$PIX rotate 359 crop 600 825 1230 550 shear $SHEAR -t$I -f white ${DATE}.jpg -o dump.jpg)
# Ensure exit code was 0, meaning OCR detected numbers of 3 digits
if [ $? -eq 0 ] && [ "${Z: -1}" != "-" ]; then
echo $Z
# Store number from OCR as integer, convert b to 6
# (as ssocr sometimes thinks 6 is B in hex)
NUMI=$(echo "$(echo $Z | sed "s/b/6/g")" | bc)
((ARRAY[$NUMI]++))
X=1
fi
done
done
done
if [ $X -eq 0 ]; then
# Failed to detect display correctly
echo ${DATE} >> bad
else
LOW=0
LVAL=-1
# Find best value out of possible candidate OCRd values
for var in "${!ARRAY[@]}";
do
# Check if this number appears the most common
if [ ${ARRAY[$var]} -gt $LOW ]; then
LOW=${ARRAY[$var]}
LVAL=$var
fi
done
# Convert number to decimal
NUM=$(echo "scale = 2; $LVAL / 10" | bc)
# Write number to CSV
echo "${DATE},$NUM" >> temps
rm ${DATE}.jpg
fi
sleep 60
done
Gnuplot file to generate graph
It is very important that you tightly crop around the digits of the display, removing the degrees Celsius notation and trying to crop above the decimal point, otherwise you will get erroneous values.
set key off
set term png transparent truecolor
set title "Fermentation Temperature" textcolor rgb "orange" font "Helvetica,50"
set object 1 rectangle from graph 0, graph 0 to graph 1, graph 1 behind fc rgbcolor 'white' fs noborder
set term png size 2000,900 font "Helvetica,30"
set xtics textcolor rgb "orange"
set ytics textcolor rgb "orange"
set output 'inkbird.png'
set datafile separator ','
set yrange [10:30]
set xdata time
set timefmt '%Y-%m-%d-%H%M'
set format x "%d %b %Y"
set xtics format "%d %b %Y %H\:%M"
set xtics rotate 90
set xtics 86400
plot 'temps.csv' using 1:2 with lines
Run Gnuplot
The awk part to strip out erroneous values, should hopefully not be necessary anymore by trying more thresholds, hopefully the right value will be obtained after OCRing and selecting.
# Target temp value set on Inkbird
# Used to strip out values less than target-7 or greater target+7
# which are likely due to incorrect OCRing
TARGET="20.0"
LTARGET=$(echo "$TARGET - 7" | bc)
HTARGET=$(echo "$TARGET + 7" | bc)
cat temps | awk -F, '{ if ($2 >= $LTARGET && $2 <= $HTARGET ) print $1 "," $2 }' > temps.csv
gnuplot temp.plt
Example image from RPi camera of Inkbird
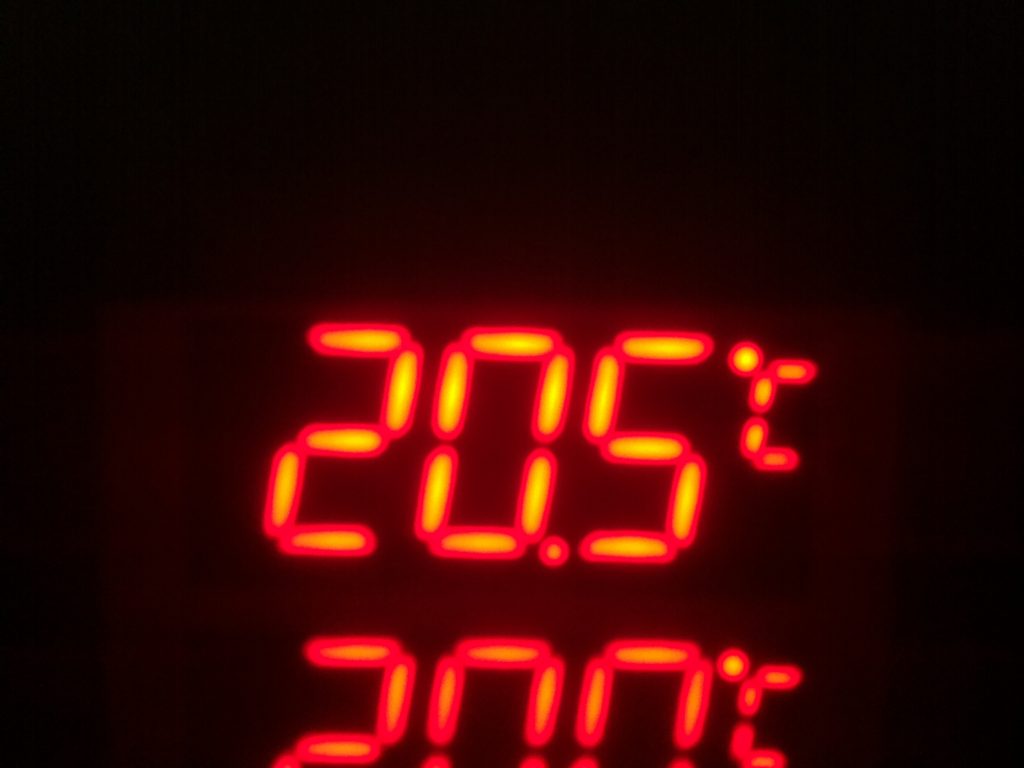
SSOCR
SSOCR can be obtained from https://github.com/auerswal/ssocr. Many thanks to Erik for producing
such an awesome program and for helping me out!.
Leave Comment
Error